Emoji picker controller with StimulusJS and Emoji Button
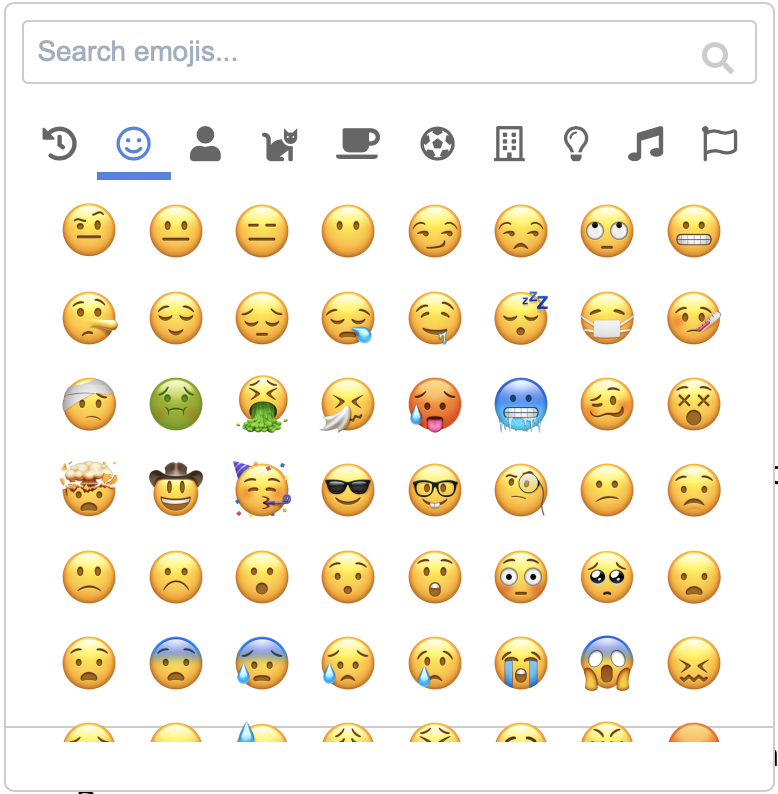
I needed an emoji picker for my latest Rails app and found Emoji Button by @joeattardi that he introduced here.
Since he already did the heavy-lifting required to provide a vanilla JavaScript emoji picker component, I just needed to add it to my app and create a Stimulus controller.
And here's how to do it for yours.
I'll assume you already have StimulusJS installed. From there, it's only a couple of steps.
First, open your Terminal and add the emoji-button package.
yarn add @joeattardi/emoji-button
Then, create a app/javascript/controllers/emoji_picker_controller.js
with this code inside
import { Controller } from "stimulus"
import EmojiButton from '@joeattardi/emoji-button'
export default class extends Controller {
static targets = [ "button", "input" ]
connect() {
this.picker = new EmojiButton()
this.picker.on('emoji', emoji => {
this.buttonTarget.innerHTML = emoji
this.inputTarget.value = emoji
})
}
toggle(event) {
event.preventDefault()
this.picker.togglePicker(event.target)
}
}
And now, if you have a Post
model with an emoji
attribute, you can do this in your form (the syntax below uses Slim instead of ERB):
= form_for post, url: post_path(post), data: { controller: "emoji-picker" } do |f|
button data-action="emoji-picker#toggle" data-target="emoji-picker.button"
= post.emoji.presence || content_tag(:i, nil, class: "fad fa-smile text-gray-400")
= f.hidden_field :emoji, data: { target: "emoji-picker.input" }
The content_tag(:i, nil, class: "fad fa-smile text-gray-400")
bit uses FontAwesome to show a default gray emoji when there is none.
And that's all there is to it. Anytime you click the button, the picker will be toggled, and if you select an emoji, both the button and the hidden form input will be updated.